题外话:
本程序利用网络上发布的公共webservice endpoint
www.webxml.com.cn/WebServices/WeatherWebService.asmx
大致步骤是
1 利用soap向webservice endpoint进行请求,取回请求结果
2 把结果显示在web界面上,web界面采用
Java
+Jsp(呵呵,有点丑陋,篇幅所迫)
好,废话少说,直接进入核心程序讲解。
一 WeatherReport类
方法 1 构造soap请求(请求格式请见上面的链接),用用户输入的城市名称镶在此请求里面
- /**
- * 获取SOAP的请求头,并替换其中的标志符号为用户输入的城市
- *
- * 编写者:王景辉
- *
- * @param city
- * 用户输入的城市名称
- * @return 客户将要发送给服务器的SOAP请求
- */
- private static String getSoapRequest(String city) {
- StringBuilder sb = new StringBuilder();
- sb
- .append( "<?xml version=\"1.0\" encoding=\"utf-8\"?>"
- + "<soap:Envelope xmlns:xsi=\"http://www.w3.org/2001/XMLSchema-instance\" "
- + "xmlns:xsd=\"http://www.w3.org/2001/XMLSchema\" "
- + "xmlns:soap=\"http://schemas.xmlsoap.org/soap/envelope/\">"
- + "<soap:Body> <getWeatherbyCityName xmlns=\"http://WebXml.com.cn/\">"
- + "<theCityName>" + city
- + "</theCityName> </getWeatherbyCityName>"
- + "</soap:Body></soap:Envelope>" );
- return sb.toString();
- }
方法 2 向endpoint发送上述SOAP请求,并设置一些请求属性,返回一个服务器端的InputStream(XML文档流)
- /**
- * 用户把SOAP请求发送给服务器端,并返回服务器点返回的输入流
- *
- * 编写者:王景辉
- *
- * @param city
- * 用户输入的城市名称
- * @return 服务器端返回的输入流,供客户端读取
- * @throws Exception
- */
- private static InputStream getSoapInputStream(String city) throws Exception {
- try {
- String soap = getSoapRequest(city);
- if (soap == null ) {
- return null ;
- }
- URL url = new URL(
- "http://www.webxml.com.cn/WebServices/WeatherWebService.asmx" );
- URLConnection conn = url.openConnection();
- conn.setUseCaches( false );
- conn.setDoInput( true );
- conn.setDoOutput( true );
- conn.setRequestProperty( "Content-Length" , Integer.toString(soap
- .length()));
- conn.setRequestProperty( "Content-Type" , "text/xml; charset=utf-8" );
- conn.setRequestProperty( "SOAPAction" ,
- "http://WebXml.com.cn/getWeatherbyCityName" );
- OutputStream os = conn.getOutputStream();
- OutputStreamWriter osw = new OutputStreamWriter(os, "utf-8" );
- osw.write(soap);
- osw.flush();
- osw.close();
- InputStream is = conn.getInputStream();
- return is;
- } catch (Exception e) {
- e.printStackTrace();
- return null ;
- }
- }
方法 3 解析方法2返回的XML文档流,并用特定的符号分隔,以便我们在Jsp页面进行结果分析
- /**
- * 对服务器端返回的XML进行解析
- *
- * 编写者:王景辉
- *
- * @param city
- * 用户输入的城市名称
- * @return 字符串 用,分割
- */
- public static String getWeather(String city) {
- try {
- Document doc;
- DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
- dbf.setNamespaceAware( true );
- DocumentBuilder db = dbf.newDocumentBuilder();
- InputStream is = getSoapInputStream(city);
- doc = db.parse(is);
- NodeList nl = doc.getElementsByTagName( "string" );
- StringBuffer sb = new StringBuffer();
- for ( int count = 0 ; count < nl.getLength(); count++) {
- Node n = nl.item(count);
- if (n.getFirstChild().getNodeValue().equals( "查询结果为空!" )) {
- sb = new StringBuffer( "#" ) ;
- break ;
- }
- sb.append(n.getFirstChild().getNodeValue() + "#\n" );
- }
- is.close();
- return sb.toString();
- } catch (Exception e) {
- e.printStackTrace();
- return null ;
- }
- }
二 weatherInfo.jsp页面
核心功能是解析 方法3 所返回的字符串,向endpoint进行请求时,一个XML文档片段是
- <? xml version = "1.0" encoding = "utf-8" ?>
- < ArrayOfString xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd = "http://www.w3.org/2001/XMLSchema" xmlns = "http://WebXml.com.cn/" >
- < string > 湖南 </ string >
- < string > 长沙 </ string >
- < string > 57687 </ string >
- < string > 57687.jpg </ string >
- < string > 2007-12-26 14:35:26 </ string >
- < string > 7℃ / 6℃ </ string >
- < string > 12月26日 小雨 </ string >
- < string > 西北风 < =3级 </ string >
- < string > 7.gif </ string >
- < string > 7.gif </ string >
- < string > 今日天气实况:多云;7.4℃;风向/风力:西北风2级;空气质量:较差;紫外线强度:最弱 </ string >
- < string > 穿衣指数:感冒指数:温度较低,较易发生感冒,请适当增加衣服。体质较弱的朋友尤其应该注意防护。晨练指数:早晨天气阴沉,气温极低,请尽量避免户外晨练,若坚持户外晨练请注意保暖防冻。交通指数:中暑指数:温度不高,其他各项气象条件适宜,中暑机率极低。公园指数:天气不好,不适宜放风筝。防晒指数:属弱紫外辐射天气,长期在户外,建议涂擦SPF在8-12之间的防晒护肤品。旅行指数:阴天,缺少阳光的陪伴,加上过低的温度会给出行带来些不便,旅游指数一般,请您在旅游时注意增加衣物。 </ string >
- < string > 8℃ / 5℃ </ string >
- < string > 12月27日 小雨 </ string >
- < string > 西北风 < =3级 </ string >
- < string > 7.gif </ string >
- < string > 7.gif </ string >
- < string > 10℃ / 4℃ </ string >
- < string > 12月28日 小雨 </ string >
- < string > 西北风 < =3级 </ string >
- < string > 7.gif </ string >
- < string > 7.gif </ string >
- < string > 长沙市位于湖南省东部偏北,湘江下游和长浏盆地西缘。其地域范围为东经111°53′-114°15′,北纬27°51′-28°41′。东邻江西省宜春地区和萍乡市,南接株洲、湘潭两市,西连娄底、益阳两市,北抵岳阳、益阳两市。东西长约230公里,南北宽约88公里。全市土地面积11819.5平方公里,其中城区面积556平方公里。长沙是一座有2000余年悠久文化历史的古城,早在春秋时期,就是楚国雄踞南方的战略要地之一。汉朝的刘邦立国之后,于公元前206年改临江为长沙,并设立汉朝的属国----长沙国,自此之后,长沙开始筑建城墙,并逐渐成为兵家必争之地。长沙属亚热带季风性湿润气候。气候特征是:气候温和,降水充沛,雨热同期,四季分明。长沙市区年平均气温17.2℃,各县16.8℃-17.3℃,年积温为5457℃,市区年均降水量1361.6毫米。景观:岳麓山、桔子洲、天心阁、烈士公园、月亮岛等。 </ string >
- </ ArrayOfString >
在Jsp中解析的代码如下,基本上是对字符串的操作,截取及截取长度的控制
- //穿衣指数
- s1 = str.substring(str.indexOf( "穿衣指数:" ),str.indexOf( "穿衣指数:" )+ 4 ) ;
- s1Content = str.substring(str.indexOf( "穿衣指数:" )+ 5 ,str.indexOf( "感冒指数:" )) ;
- //感冒指数
- s2 = str.substring(str.indexOf( "感冒指数:" ),str.indexOf( "感冒指数:" )+ 4 ) ;
- s2Content = str.substring(str.indexOf( "感冒指数:" )+ 5 ,str.indexOf( "晨练指数:" )) ;
- //晨练指数
- s3 = str.substring(str.indexOf( "晨练指数:" ),str.indexOf( "晨练指数:" )+ 4 ) ;
- s3Content = str.substring(str.indexOf( "晨练指数:" )+ 5 ,str.indexOf( "交通指数:" )) ;
- //交通指数
- s7 = str.substring(str.indexOf( "交通指数:" ),str.indexOf( "交通指数:" )+ 4 ) ;
- s7Content = str.substring(str.indexOf( "交通指数:" )+ 5 ,str.indexOf( "中暑指数:" )) ;
- //中暑指数
- s4 = str.substring(str.indexOf( "中暑指数:" ),str.indexOf( "中暑指数:" )+ 4 ) ;
- s4Content = str.substring(str.indexOf( "中暑指数:" )+ 5 ,str.indexOf( "防晒指数:" )) ;
- //防晒指数
- s5 = str.substring(str.indexOf( "防晒指数:" ),str.indexOf( "防晒指数:" )+ 4 ) ;
- s5Content = str.substring(str.indexOf( "防晒指数:" )+ 5 ,str.indexOf( "旅行指数:" )) ;
- //旅行指数
- s6 = str.substring(str.indexOf( "旅行指数:" ),str.indexOf( "旅行指数:" )+ 4 ) ;
- s6Content = str.substring(str.indexOf( "旅行指数:" )+ 5 ) ;
程序运行效果见附件上的截图!!!运行附件: http://localhost:8080/yourProject/tianqi.jsp
好了,基本上核心代码就是上边那些了!不仅如此,加入我们想要在自己的系统里加入飞机票,火车票,股票信息等等之类的功能,只要有相应的webservice,我们都可以实现(呵呵,好像免费的少哦),各位有什么疑问,留言吧!!!
- 天气预报源程序.rar (265.2 KB)
- 描述: 源程序
- 下载次数: 719
更多文章、技术交流、商务合作、联系博主
微信扫码或搜索:z360901061
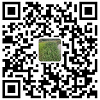
微信扫一扫加我为好友
QQ号联系: 360901061
您的支持是博主写作最大的动力,如果您喜欢我的文章,感觉我的文章对您有帮助,请用微信扫描下面二维码支持博主2元、5元、10元、20元等您想捐的金额吧,狠狠点击下面给点支持吧,站长非常感激您!手机微信长按不能支付解决办法:请将微信支付二维码保存到相册,切换到微信,然后点击微信右上角扫一扫功能,选择支付二维码完成支付。
【本文对您有帮助就好】元
